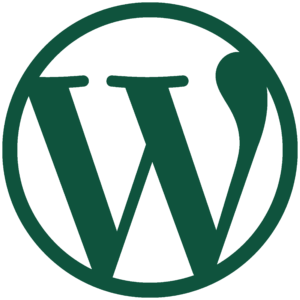
There are a few functions available to retrieve the URL and size of an image in the WordPress Media Library, but few people know that these functions will often lie about an image’s dimensions.
As an example, let’s define a custom image size of 1000 x 1000 cropped, and use image_downsize()
to retrieve the URL and size of an image ID (this example can be used with wp_get_attachment_image_src()
as well). I’ll use list()
in the example, instead of an array variable for the return values, to keep the code more readable. ;-)
add_image_size( 'my-custom-size', 1000, 1000, true );
list( $img_url, $img_width, $img_height, $img_is_intermediate ) = image_downsize( $id, 'my-custom-size' );
WordPress will return a URL for the image and the values of $width / $height may be 1000 / 1000 – it all depends on several factors.