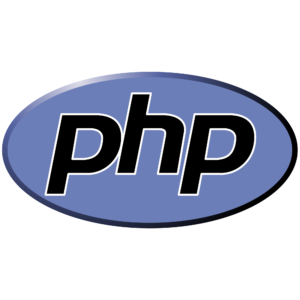
The WordPress remove_action()
and remove_filter()
functions are useful, but they require the original hook priority and a function name, a class object and method name, or a class name and static method name.
Providing the class object in particular may be difficult or impossible, as plugin and theme classes may be extended and instantiated any number of ways. The following SucomUtilWP::remove_filter_hook_name()
static method can be used to remove WordPress action and filter hooks using their class name instead of requiring a class object.
For example, if an action hook like this one has been added within a class object that was not saved, saved in a private/protected variable, or cannot be re-instantiated, then removing it might be impossible.
add_action( 'action_name', array( $this, 'method_name' ), 1000, 2 );
If you know the class (or extended class) name (‘Plugin_Namespace\Class_Name’ for this example), you can remove it using the following static method:
SucomUtilWP::remove_filter_hook_name( 'action_name', 'Plugin_Namespace\Class_Name::method_name' );
The SucomUtilWP::remove_filter_hook_name()
static method:
<?php
if ( ! class_exists( 'SucomUtilWP' ) ) {
class SucomUtilWP {
/*
* Filters and actions are both saved in the $wp_filter global variable.
*/
public static function remove_action_hook_name( $filter_name, $hook_name ) {
self::remove_filter_hook_name( $filter_name, $hook_name );
}
/*
* Loop through all action/filter hooks and remove any that match the given function or static method name.
*
* Note that class object methods are matched using a class static method name.
*/
public static function remove_filter_hook_name( $filter_name, $hook_name ) {
global $wp_filter;
if ( isset( $wp_filter[ $filter_name ]->callbacks ) ) {
foreach ( $wp_filter[ $filter_name ]->callbacks as $hook_prio => $hook_group ) {
foreach ( $hook_group as $hook_id => $hook_info ) {
/*
* Returns a function name or a class static method name.
*
* Class object methods are returned as class static method names.
*/
if ( self::get_hook_function_name( $hook_info ) === $hook_name ) {
unset( $wp_filter[ $filter_name ]->callbacks[ $hook_prio ][ $hook_id ] );
}
}
}
}
}
/*
* Returns a function name or a class static method name.
*
* Class object methods are returned as class static method names.
*/
public static function get_hook_function_name( array $hook_info ) {
$hook_name = '';
if ( isset( $hook_info[ 'function' ] ) ) {
/*
* The callback hook is a dynamic or static method.
*/
if ( is_array( $hook_info[ 'function' ] ) ) {
$class_name = '';
$function_name = '';
/*
* The callback hook is a dynamic method.
*/
if ( is_object( $hook_info[ 'function' ][ 0 ] ) ) {
$class_name = get_class( $hook_info[ 'function' ][ 0 ] );
/*
* The callback hook is a static method.
*/
} elseif ( is_string( $hook_info[ 'function' ][ 0 ] ) ) {
$class_name = $hook_info[ 'function' ][ 0 ];
}
if ( is_string( $hook_info[ 'function' ][ 1 ] ) ) {
$function_name = $hook_info[ 'function' ][ 1 ];
}
/*
* Return a static method name.
*/
$hook_name = $class_name . '::' . $function_name;
/*
* The callback hook is a function.
*/
} elseif ( is_string( $hook_info[ 'function' ] ) ) {
$hook_name = $hook_info[ 'function' ];
}
}
return $hook_name;
}
}
}