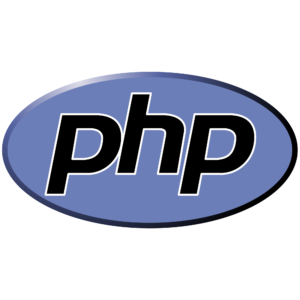
The WordPress remove_action()
and remove_filter()
functions are useful, but they require the original hook priority and a function name, a class object and method name, or a class name and static method name.
Providing the class object in particular may be difficult or impossible, as plugin and theme classes may be extended and instantiated any number of ways. The following SucomUtilWP::remove_filter_hook_name()
static method can be used to remove WordPress action and filter hooks using their class name instead of requiring a class object.
For example, if an action hook like this one has been added within a class object that was not saved, saved in a private/protected variable, or cannot be re-instantiated, then removing it might be impossible.
add_action( 'action_name', array( $this, 'method_name' ), 1000, 2 );
If you know the class (or extended class) name (‘Plugin_Namespace\Class_Name’ for this example), you can remove it using the following static method:
SucomUtilWP::remove_filter_hook_name( 'action_name', 'Plugin_Namespace\Class_Name::method_name' );